Documentation
Welcome to the Py-Engineering documentation. If you're new to these docs, we recommend you to check what Py-Engineering is all about. Watch the introduction movie "How it works" and checkout some examples. If you think anything is missing or unclear, let us know. Ready to start, but no Py-Engineering account yet? Request a demo!
Getting started
No need to install anything. Py-Engineering is a web-application. You only need an account and/or an API key. If you don’t have one, reach out to someone in your company who can provide you one or request a demo by mailing to info@py-engineering.com.
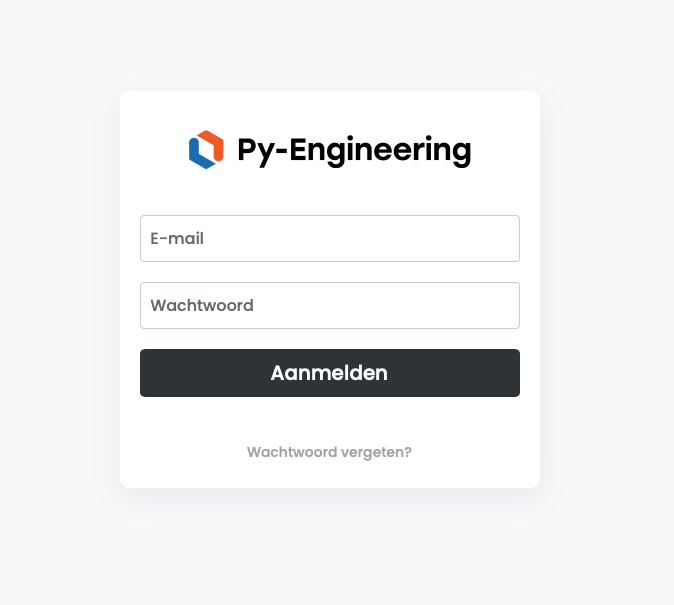
Example of a login page on py-engineering.com. Depending on the platform your account belongs to, this login page might have a different URL and look-and-feel.
How it works
The general concept is that you write a Python function that prints output like you would do on your local machine. By defining input variables in Py-Engineering.com, this function is instantly accessible as web-app or API. By running the tool in the web-app or trough the API, the Python function is executed in our cloud environment. See what this looks like in the introduction video below or check out the Tutorials.
Platform settings
Easily configure platform-wide options for a consistent user experience.
General platform settings
The slug defines the structure of the platform's URLs. It determines where the platform and its related pages can be accessed. For example, if the slug is set to demo
, the following URLs will be used:
- Platform home page: https://py-engineering.com/demo
- Login page: https://py-engineering.com/demo/login
- Registration page (if platform is open for signup): https://py-engineering.com/platform_signup/demo
The Name of the platform is displayed on the home page if no logo is provided.
Created tools can support multiple languages based on the selected Languages.
For tools that require an account, Email verification and Multi-Factor Authentication (MFA) can be configured as mandatory.
By default, all platform pages display the Py-Engineering logo at the bottom. This can be disabled by setting Show Py-Engineering logo to false
.
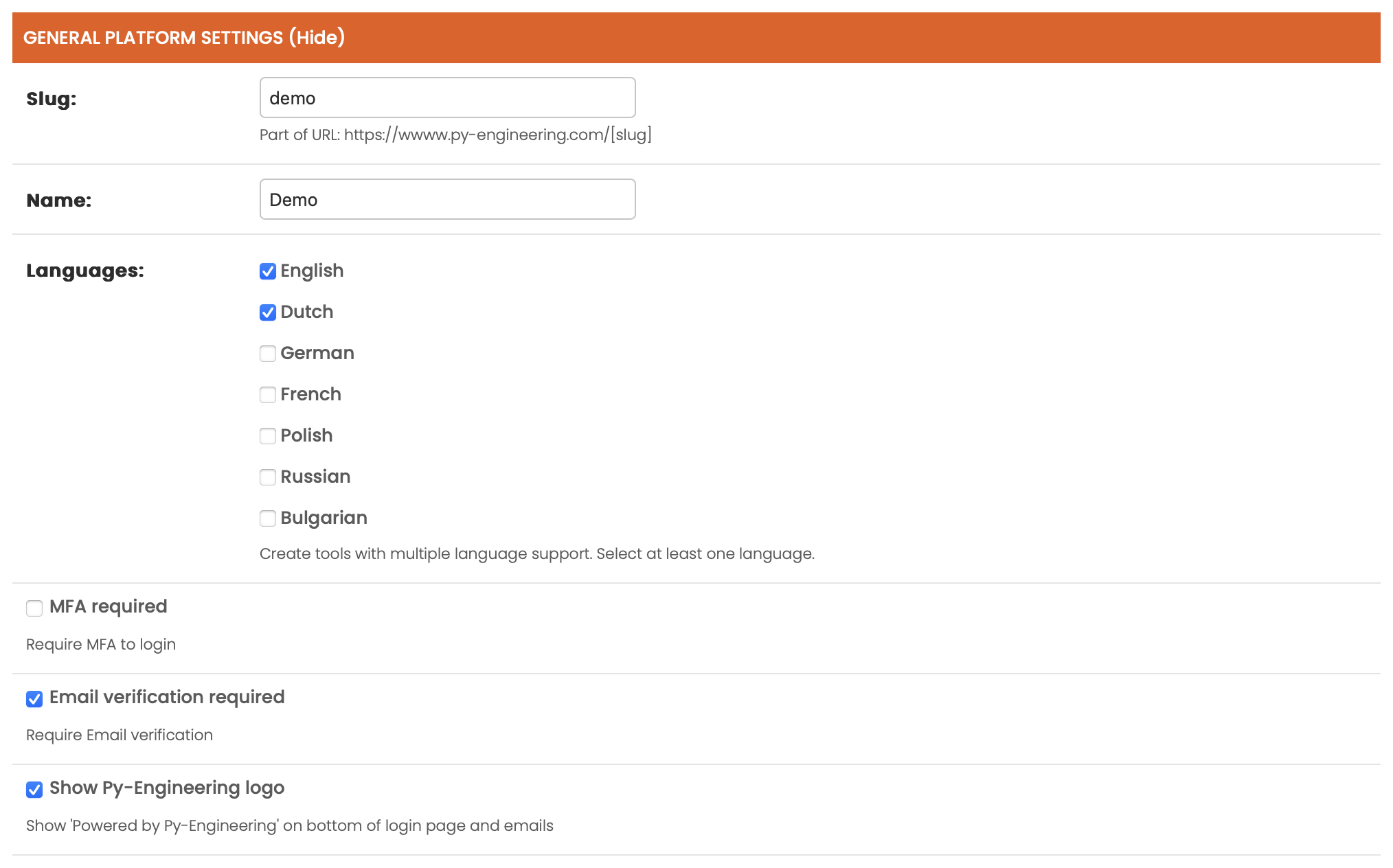
Lay out
The Primary color defines the main accent color of the platform, which is used for buttons, links, and other highlighted elements.
The Secondary color is used for complementary elements, such as backgrounds and less prominent UI components.
The Logo is displayed on the home page and throughout the platform. If no logo is provided, the platform name will be shown instead.
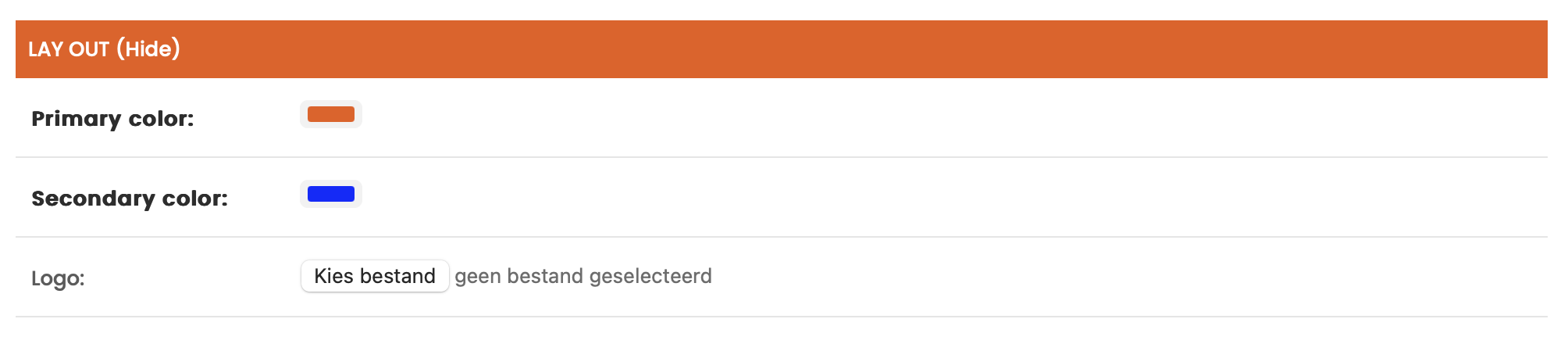
Signup
The Allow sign up option determines whether users can create an account on the platform. If disabled, only existing users can log in.
The Company name in signup form checkbox adds a field for users to enter their company name during registration.
The Phone number in signup form checkbox adds a field for users to provide their phone number when signing up.
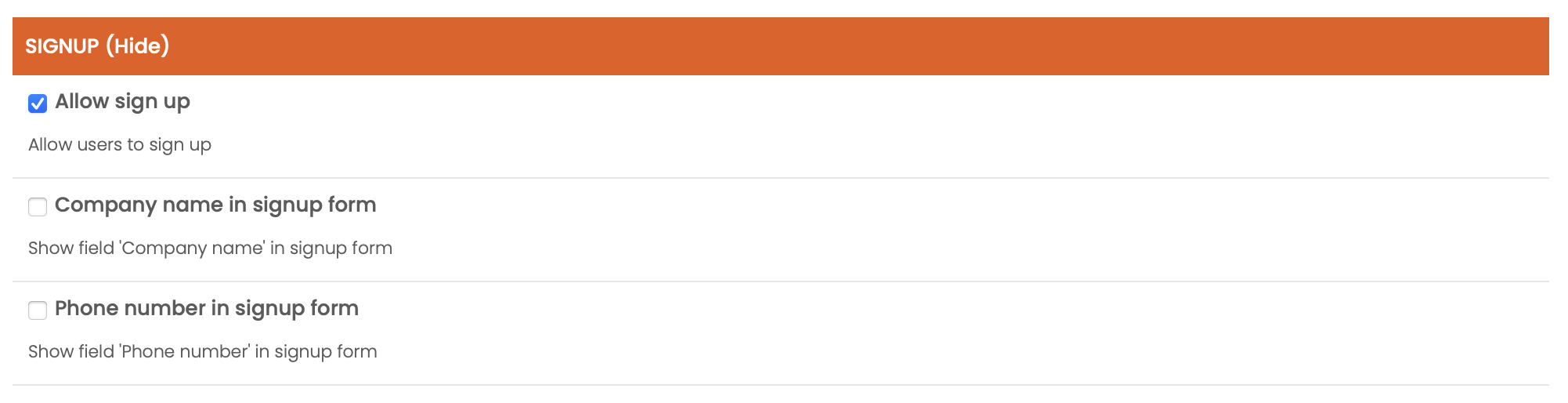
The image below shows a signup form with the Company Name and Phone Number fields enabled. When these options are selected, users are required to enter this information during registration.
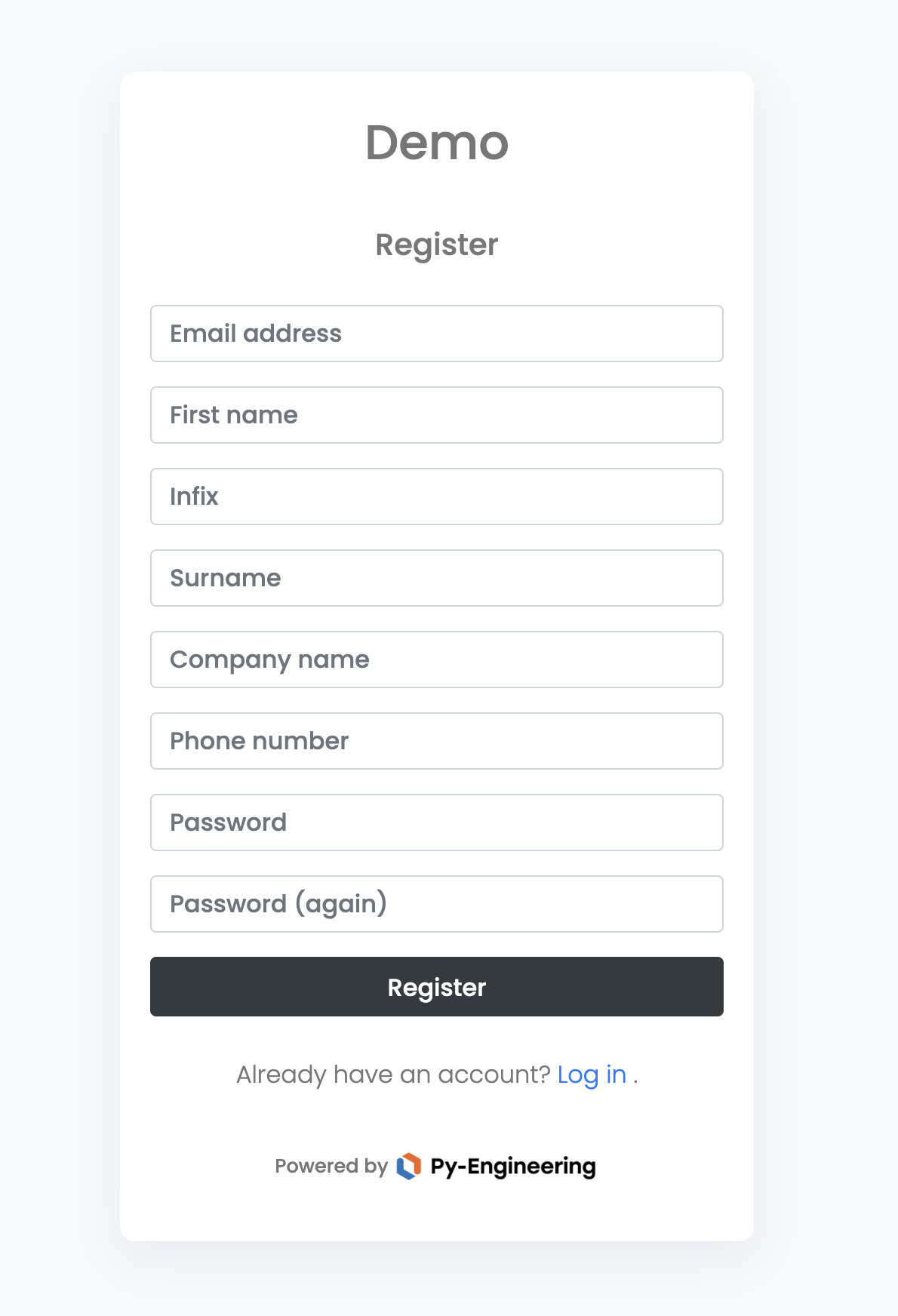
Connectivity
By default, emails are sent from noreply@py-engineering.com
. If you wish to send emails from a different address, you can configure a Mail server.
The Domain setting allows the platform to be hosted on a custom domain instead of py-engineering.com
. If a custom domain is used, a DNS server must be configured in coordination with the Py-Engineering developers.
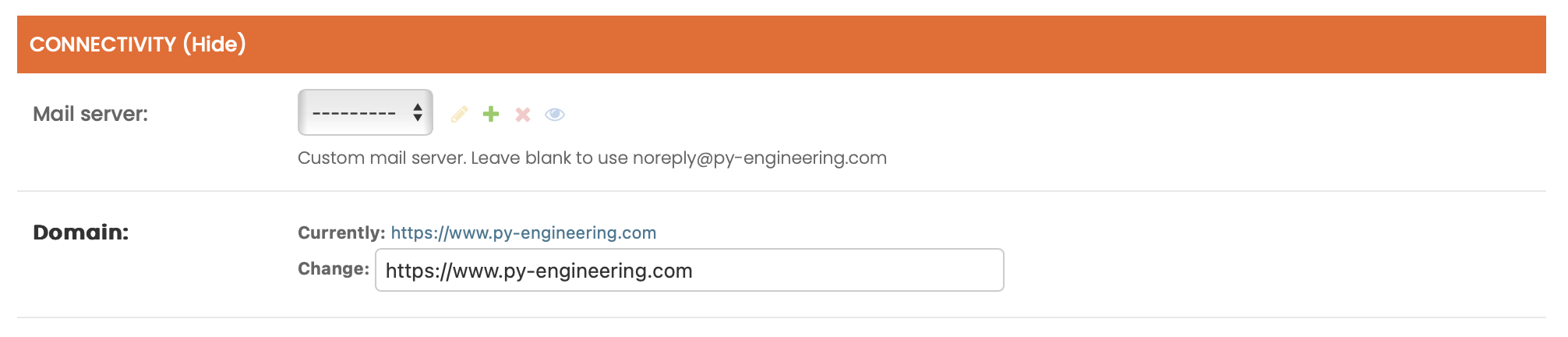
The SMTP settings can be configured via Platform > Mail server or by clicking the (+) directly
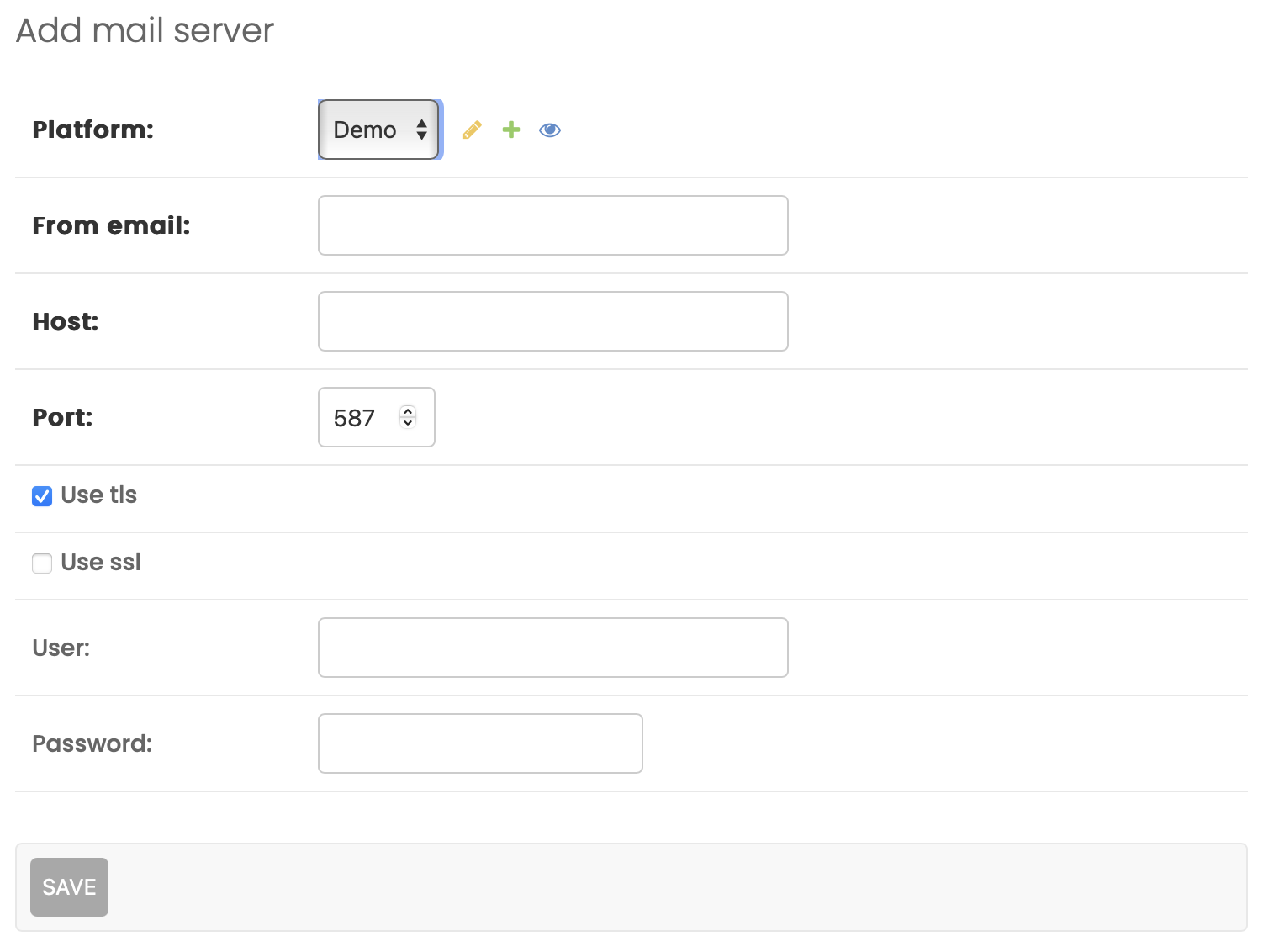
Tool settings
Define the behavior and properties of each tool directly within your Python scripts. Control how your tools are displayed and interacted with by users.
General tool settings
Slug defines the url of the tool. A tool can be found on the platform page https://py-engineering.com/demo/tool-a if slug is tool-a.
The Name of the platform is the name as shown on the tool page.
If multliple Languages are selected, multilanguage support becomes active. After saving the page, fields such as Name are given multiple input elements for each selected language. In the GUI, a dropdown for the selected language is shown in the upper right menu.
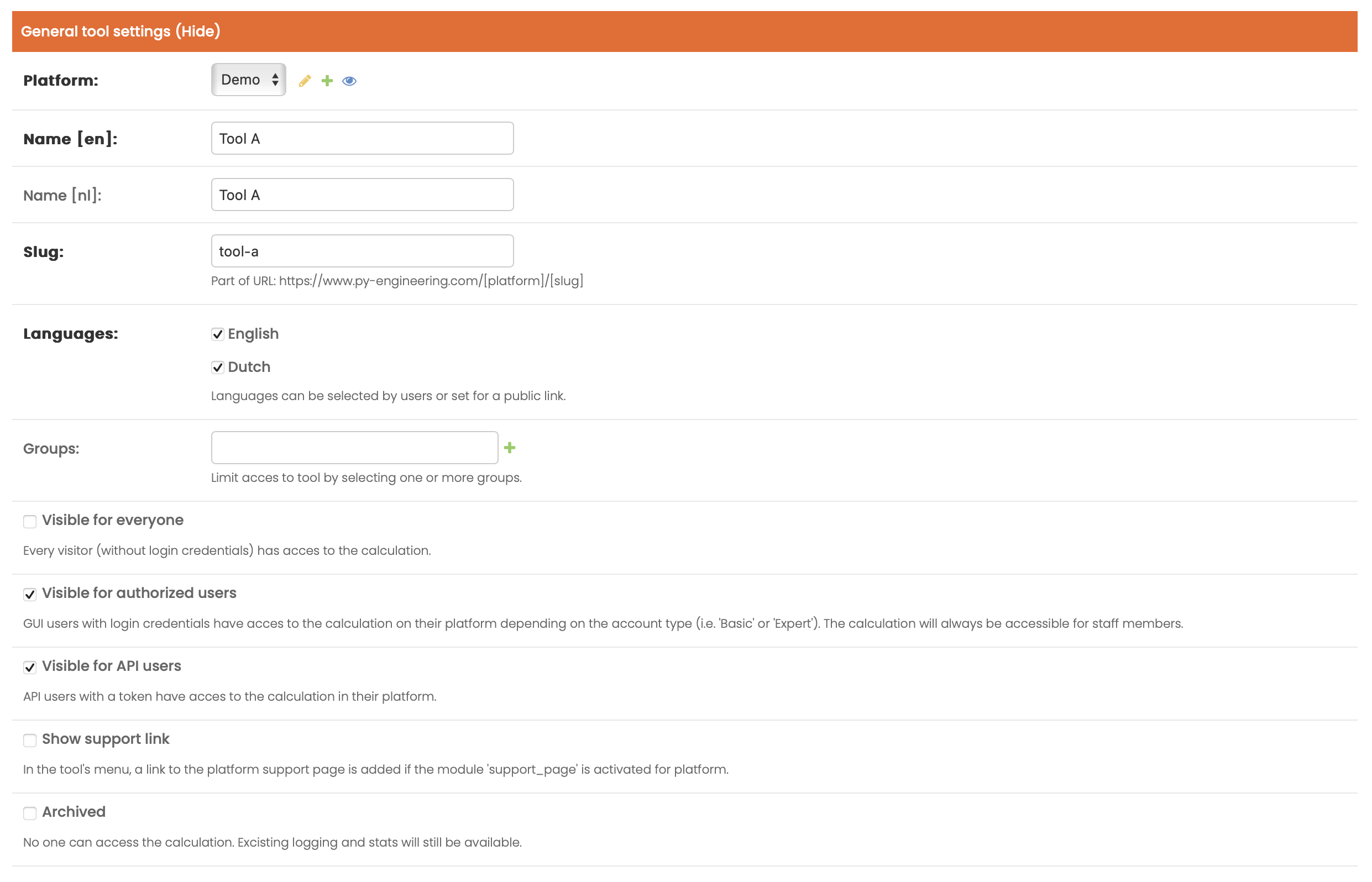
Output
All statements printed by the main Python function are treated as output.
Output tags
To enhance the presentation, specific tags can be used to format the output. These tags begin with an underscore (_
) and apply to the text either on the same line or across multiple lines enclosed within square brackets ([ ]
).
Tag | HTML Substitution |
---|---|
_BOLD | <b>{}</b> |
_ITALIC | <i>{}</i> |
_UNDERLINE | <u>{}</u> |
_SUPERSCRIPT | <sup>{}</sup> |
_SUBSCRIPT | <sub>{}</sub> |
_CODE | <code>{}</code> |
_PREFORMATTED | <pre>{}</pre> |
_INFO | <p class="text-info my-1"><span class="fa fa-fw fa-info-circle"></span> {}</p> |
_WARNING | <p class="text-warning my-1"><span class="fa fa-fw fa-exclamation-circle"></span> {}</p> |
_DANGER | <p class="text-danger my-1"><span class="fa fa-fw fa-times-circle"></span> {}</p> |
_SUCCESS | <p class="text-success my-1"><span class="fa fa-fw fa-check-circle"></span> {}</p> |
_HEADER | <h6 class="pt-2">{}</h6> |
_SMALL | <small>{}</small> |
_HR | <hr>{} |
An example:
def main_function(input_dict):
# Single line, starting a tag
print('_HEADER Header')
print('The rest of this line _UNDERLINE will be underlined on a new line.')
for tag in ['INFO', 'WARNING', 'DANGER', 'SUCCESS']:
print(f"_{tag} {tag.title()}")
# Inline with [..]
print('_BOLD[This] is bold and _ITALIC[this] is italic.')
print('The tags _BOLD[_ITALIC[_UNDERLINE[can be combined]]]')
# Multiple lines with [..]
print('_PREFORMATTED[')
print('Multiple lines')
print('can be formatted')
print('using brackets [ and _]')
print('_SMALL Note that an endbracket within a tag needs to be escaped with a _CODE[ _ ]')
print(']')
This will result in the following output on the tool page:
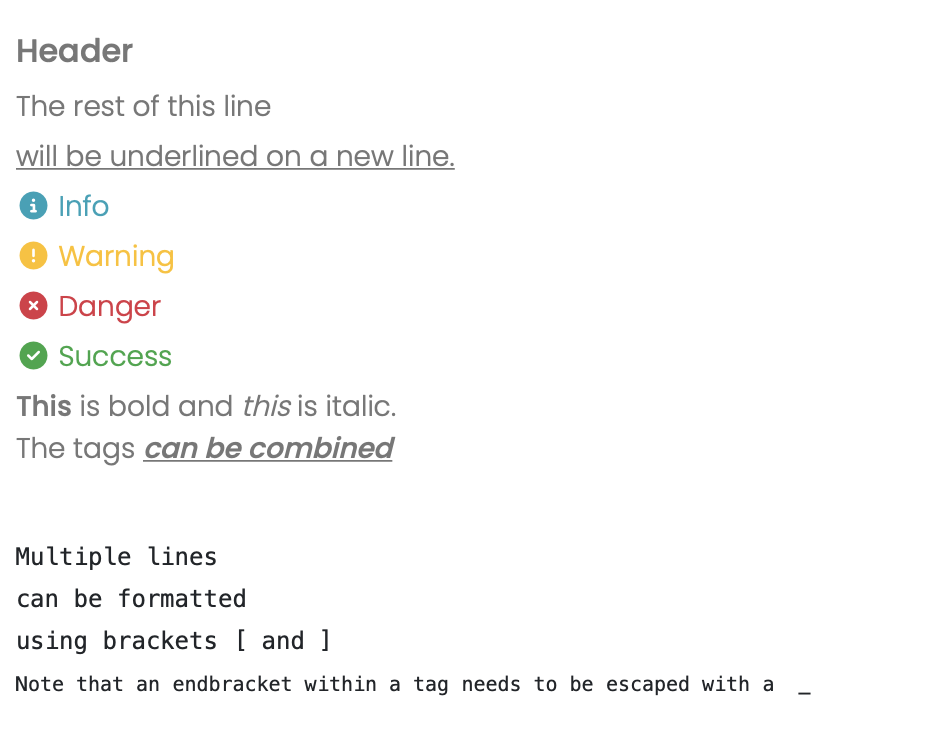
Create links and buttons
Just like output tags, the _IMAGE
, _DOWNLOAD_LINK
and _DOWNLOAD_BUTTON
tags can be used to generate visually appealing links and buttons for files or URLs.
Tag | HTML Substitution |
---|---|
_IMAGE | <img src="{file_url}" alt="{file_name}" class="img-fluid"><br> |
_DOWNLOAD_LINK | <a href="{}">{}</a> |
_DOWNLOAD_BUTTON | <a href="{}" class="btn btn-outline-secondary btn-sm"><span class="fa-fw fal fa fa-download"></span> {}</a><br> |
An example:
import xlsxwriter
import matplotlib.pyplot as plt
def main_function(input_dict):
file_name = 'example'
fig, ax = plt.subplots()
fruits = ['apple', 'blueberry', 'cherry', 'orange']
counts = [40, 100, 30, 55]
bar_labels = ['red', 'blue', '_red', 'orange']
bar_colors = ['tab:red', 'tab:blue', 'tab:red', 'tab:orange']
ax.bar(fruits, counts, label=bar_labels, color=bar_colors)
ax.set_ylabel('fruit supply')
ax.set_title('Fruit supply by kind and color')
ax.legend(title='Fruit color')
plt.savefig(file_name)
workbook = xlsxwriter.Workbook(file_name)
worksheet = workbook.add_worksheet()
worksheet.write('A1', 'Hello world')
workbook.close()
print("Show image:")
print(f"_IMAGE[{file_name}.png]")
print(f"Use a link like _DOWNLOAD_LINK[{file_name}.xlsx] to download a file. Or create a button:")
print(f"\n_DOWNLOAD_BUTTON[{file_name}.xlsx]")
This will provide the following image, link and download button:
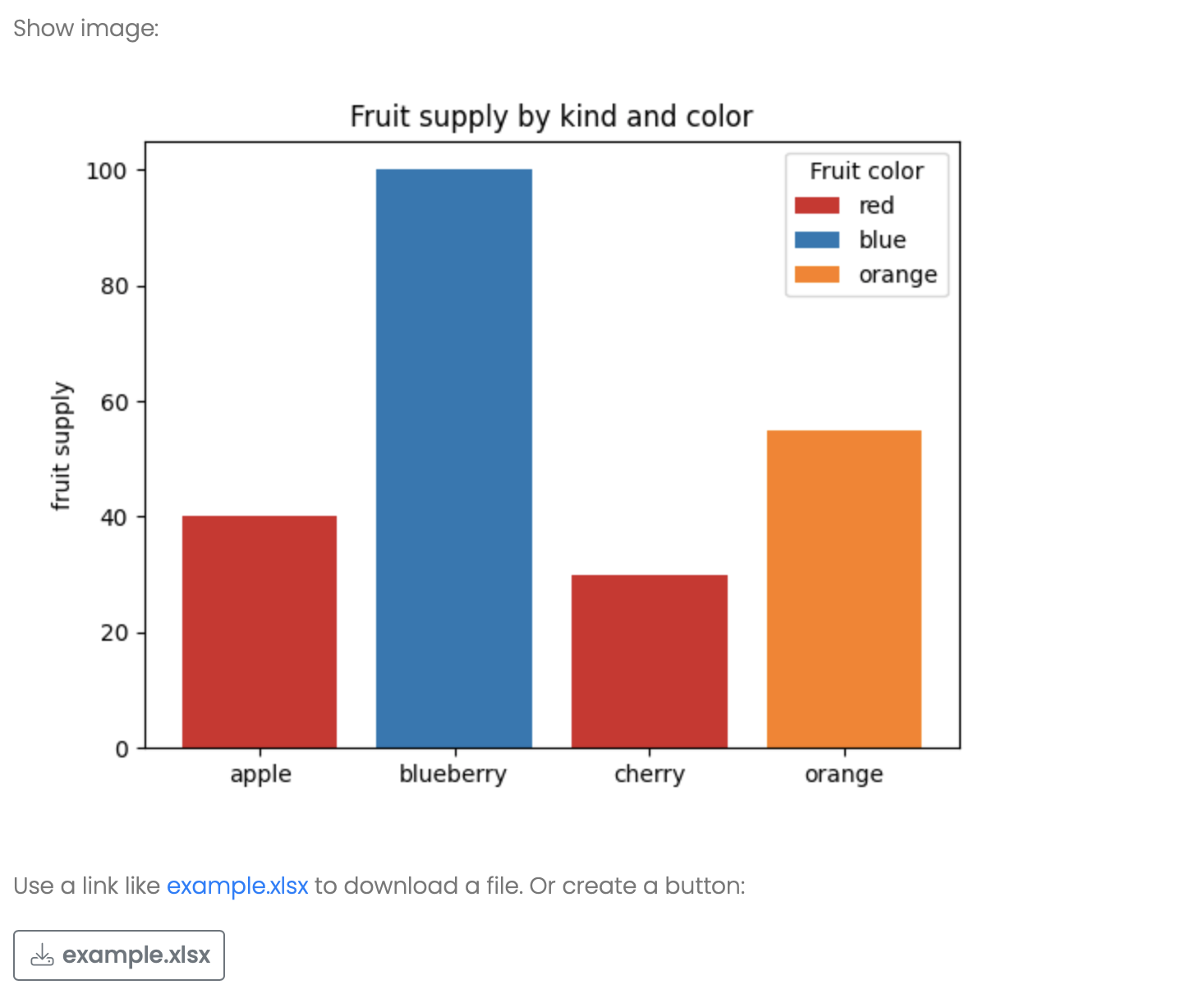
Upload data
Upload files like TXT, CSV or XLSX that can be used in one or more tools.
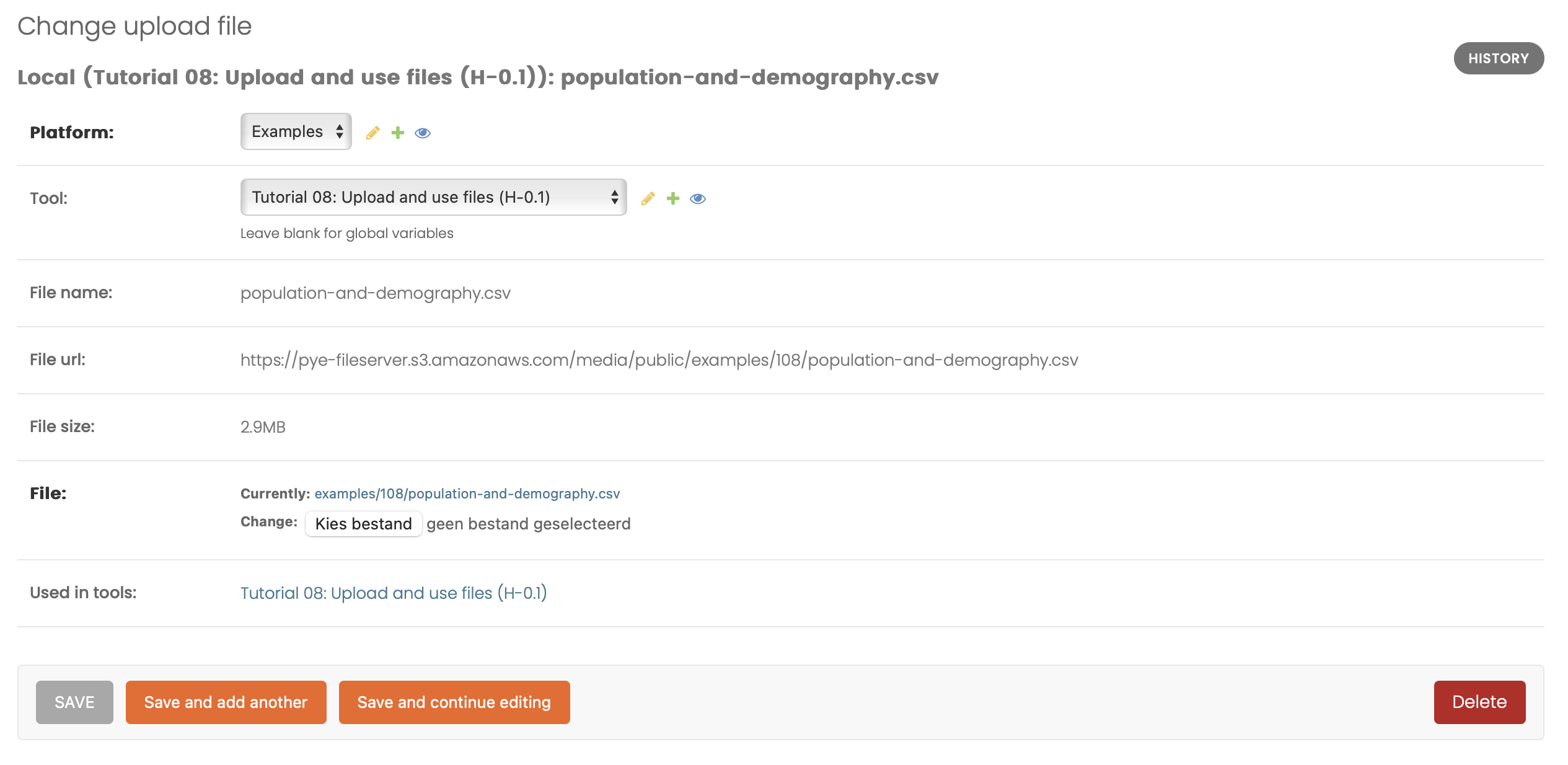
Sharing
Control access and share your tools with just a few clicks.
Users and authorization
Control who can view, edit, or use tools with fine-grained authorization settings.
Monitoring
Keep track of tool usage and platform activity with real-time logging and statistics.
API
Integrate the tools as an API into your workflow with minimal effort using clear documentation and real-world examples.